So guys in today’s blog we will see how to Blur Faces in Live Feed using OpenCV and Python. This is going to be a very interesting blog, so without any further due, let’s do it…
Snapshot of our Final Result…
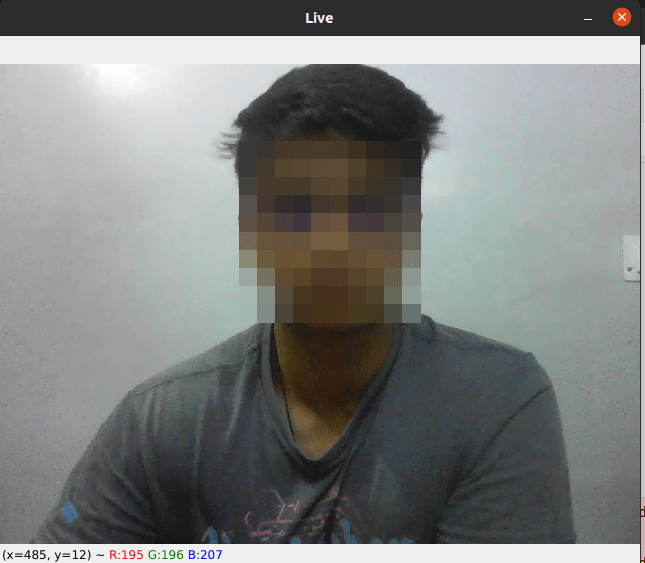
Step 1 – Importing required libraries
import cv2 import numpy as np
Step 2 – Defining the Blur function
- Here we are defining the Blur function.
- It takes 2 arguments, the image and the blur factor (k).
- Then we are simply calculating the kernel height and kernel width by dividing the height and width by the blur factor. The smaller the kw and kh, the higher the blurring will be.
- Then we are simply checking if kw and kh are odd or not, if they are even then simply subtract 1 from them to make them odd.
- Then simply we are applying the Gaussian Blur to our Image and return it. We can also apply any other Blur operations, read more about them here.
def blur(img,k): h,w = img.shape[:2] kh,kw = h//k,w//k if kh%2==0: kh-=1 if kw%2==0: kw-=1 img = cv2.GaussianBlur(img,ksize=(kh,kw),sigmaX=0) return img
Step 3 – Defining pixelate_face function
- This is a function that simply adds a pixelated effect to blurred Images.
def pixelate_face(image, blocks=10): # divide the input image into NxN blocks (h, w) = image.shape[:2] xSteps = np.linspace(0, w, blocks + 1, dtype="int") ySteps = np.linspace(0, h, blocks + 1, dtype="int") # loop over the blocks in both the x and y direction for i in range(1, len(ySteps)): for j in range(1, len(xSteps)): # compute the starting and ending (x, y)-coordinates # for the current block startX = xSteps[j - 1] startY = ySteps[i - 1] endX = xSteps[j] endY = ySteps[i] # extract the ROI using NumPy array slicing, compute the # mean of the ROI, and then draw a rectangle with the # mean RGB values over the ROI in the original image roi = image[startY:endY, startX:endX] (B, G, R) = [int(x) for x in cv2.mean(roi)[:3]] cv2.rectangle(image, (startX, startY), (endX, endY), (B, G, R), -1) # return the pixelated blurred image return image
Step 4 – Let’s Blur faces in the Live feed
- The code below is the main part to run the code.
- The factor here defines the amount of blurring.
- Defining a cascade Classifier object to detect faces.
- Download the haarcascade_frontalface_default.xml file.
- Then simply run an infinite loop that reads the images from the webcam, detects faces in them, and then replaces that face part with pixelated faces.
- Read more about Face and Eye Detection using HAARCASCADES.
factor = 3 cap = cv2.VideoCapture(0) face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') while 1: ret,frame = cap.read() gray = cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY) faces = face_cascade.detectMultiScale(gray, 1.5, 5) for (x,y,w,h) in faces: frame[y:y+h,x:x+w] = pixelate_face(blur(frame[y:y+h,x:x+w],factor)) cv2.imshow('Live',frame) if cv2.waitKey(1)==27: break cap.release() cv2.destroyAllWindows()
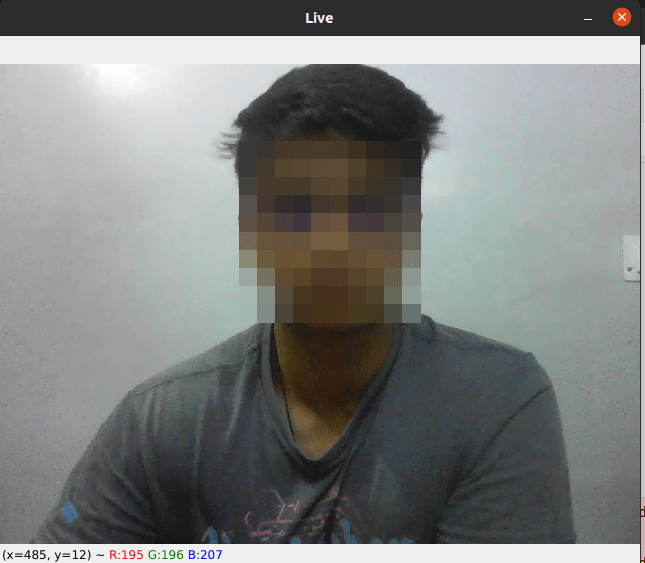
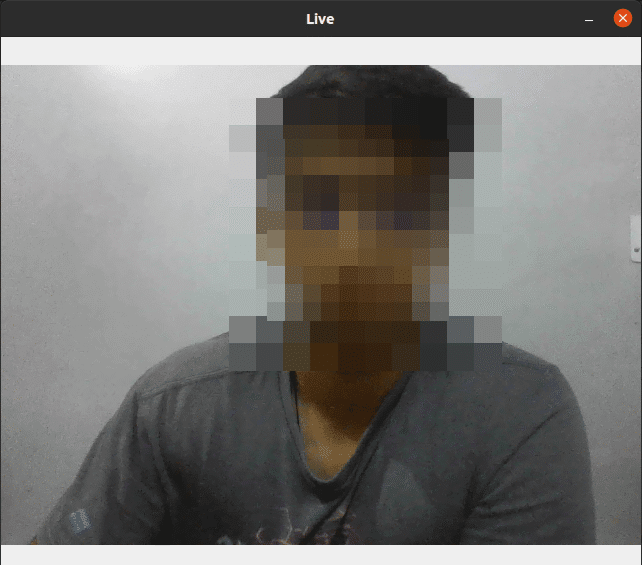
Let’s see the whole code…
import cv2 import numpy as np def blur(img,k): h,w = img.shape[:2] kh,kw = h//k,w//k if kh%2==0: kh-=1 if kw%2==0: kw-=1 img = cv2.GaussianBlur(img,ksize=(kh,kw),sigmaX=0) return img def pixelate_face(image, blocks=10): # divide the input image into NxN blocks (h, w) = image.shape[:2] xSteps = np.linspace(0, w, blocks + 1, dtype="int") ySteps = np.linspace(0, h, blocks + 1, dtype="int") # loop over the blocks in both the x and y direction for i in range(1, len(ySteps)): for j in range(1, len(xSteps)): # compute the starting and ending (x, y)-coordinates # for the current block startX = xSteps[j - 1] startY = ySteps[i - 1] endX = xSteps[j] endY = ySteps[i] # extract the ROI using NumPy array slicing, compute the # mean of the ROI, and then draw a rectangle with the # mean RGB values over the ROI in the original image roi = image[startY:endY, startX:endX] (B, G, R) = [int(x) for x in cv2.mean(roi)[:3]] cv2.rectangle(image, (startX, startY), (endX, endY), (B, G, R), -1) # return the pixelated blurred image return image factor = 3 cap = cv2.VideoCapture(0) face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml') while 1: ret,frame = cap.read() gray = cv2.cvtColor(frame,cv2.COLOR_BGR2GRAY) faces = face_cascade.detectMultiScale(gray, 1.5, 5) for (x,y,w,h) in faces: frame[y:y+h,x:x+w] = pixelate_face(blur(frame[y:y+h,x:x+w],factor)) cv2.imshow('Live',frame) if cv2.waitKey(1)==27: break cap.release() cv2.destroyAllWindows()
And this is how you Blur Faces in Live Feed…
So this is all for this blog folks. Thanks for reading it and I hope you are taking something with you after reading this and till the next time …
Read my previous post: How to Extract Tables from PDF files and save them as CSV using Python
Check out my other machine learning projects, deep learning projects, computer vision projects, NLP projects, and Flask projects at machinelearningprojects.net.