Hey guys in today’s blog we will see how you can create your first web app using Flask in Python. This is going to be a very interesting blog, so without any further due, let’s do it…
Are you interested in creating your own web application but unsure where to start? Look no further! In this comprehensive guide, we will walk you through the process of building your first web app using Flask, a powerful and beginner-friendly Python web framework. Flask enables you to develop web applications quickly and efficiently with minimal code. Let’s dive in and begin constructing your very own web app!
Checkout the video here – https://youtu.be/Vvf_mGajUUs
Step 0: Understanding Flask
Before we embark on this journey, let’s familiarize ourselves with Flask. Flask is a lightweight web framework written in Python. It offers essential tools and libraries for developing web applications, making it an excellent choice for beginners. Flask follows a “micro” design philosophy, prioritizing simplicity and extensibility, allowing you to add only the necessary features.
Step 1: Installing and Setting Up Flask
To get started, ensure that Python is installed on your computer. Once you have Python, installing Flask is a breeze using pip, the Python package installer. Open your terminal or command prompt and enter the following command:
pip install flask
This command will download and install Flask on your system.
Step 2: Create a directory to work in
With Flask installed, let’s create a new directory for your project.
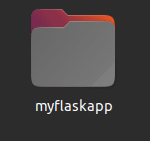
Now create the following 2 things in the folder:
- A file called app.py. This file will serve as the entry point for your Flask application.
- A folder called templates
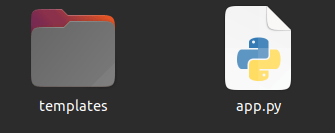
Step 3: Coding Your Flask Web App
In the app.py
file, begin by importing the Flask module and creating a Flask instance:
from flask import Flask,render_template app = Flask(__name__) @app.route('/') def home(): return "Welcome to my Flask web app!"
Subsequently, define a route that corresponds to the root URL of your web app. This route will handle incoming requests to your application:
Congratulations! You have just established your first Flask route. When users visit the root URL of your web app, they will be greeted with the message, “Welcome to my Flask web app!”
Step 4: Running Your Web App
To run your web app, return to your terminal or command prompt, navigate to the directory where your app.py
file is located, and execute any of the following commands:
Command 1
python app.py
OR
Command 2
flask run
You should see output similar to the following:
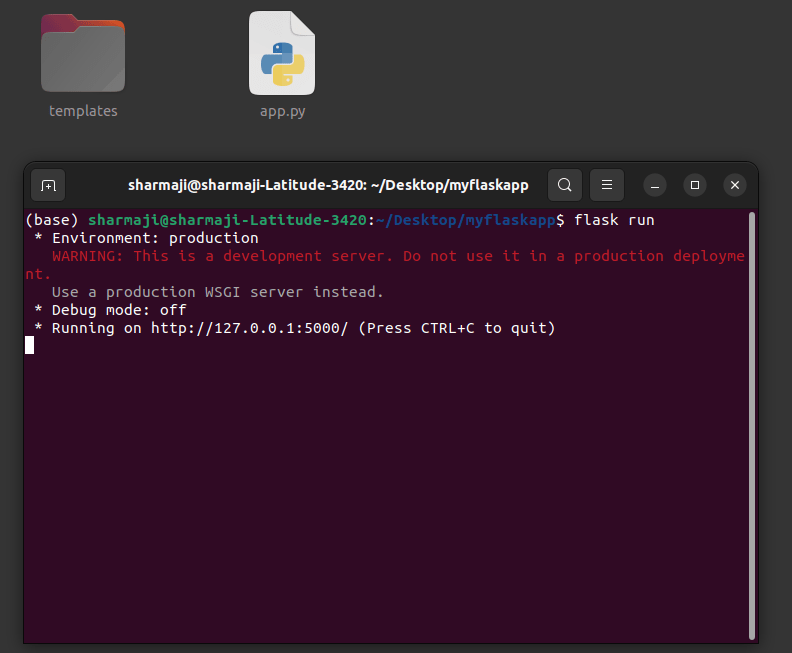
Open your web browser and visit http://127.0.0.1:5000/
. You should now observe the message you defined in the home()
function.
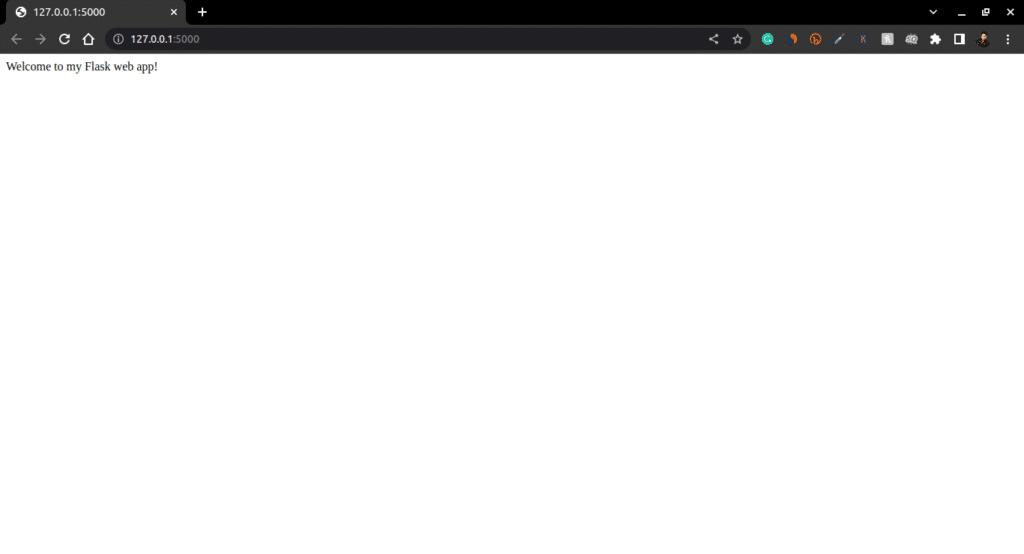
Step 5: Expanding Your Web App
Now that you have the basic structure of your web app, it’s time to enhance its functionality. Flask enables you to define additional routes and handle different URLs.
For instance, you can create a new route that displays an “About” page:
from flask import Flask,render_template app = Flask(__name__) @app.route('/') def home(): return "Welcome to my Flask web app!" @app.route('/about') def about(): return "This is my Flask web app!"
By visiting http://127.0.0.1:5000/about
, users will encounter the message, “This is my Flask web app!”
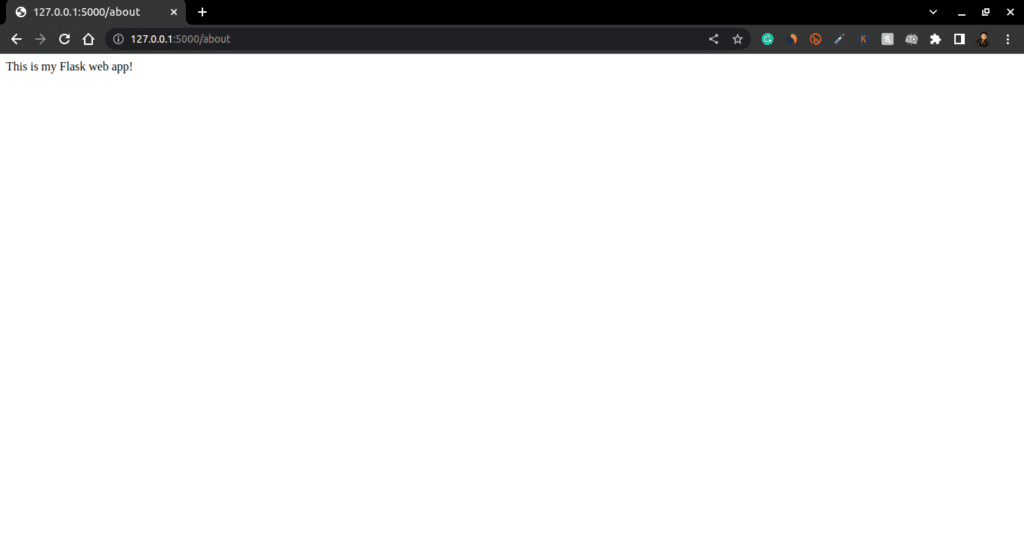
Step 6: Templating and Styling
Flask supports templates, which allow you to separate the structure of your web app from its content. HTML templates enable the creation of dynamic web pages.
Within your project directory, create a new directory called templates
. Inside the templates
directory, create a new file named index.html
. Add the following content to index.html
:
<!DOCTYPE html> <html> <head> <title>My Flask Web App</title> </head> <body> <h1>Welcome to my Flask web app!</h1> <p>This is a sample web page.</p> </body> </html>
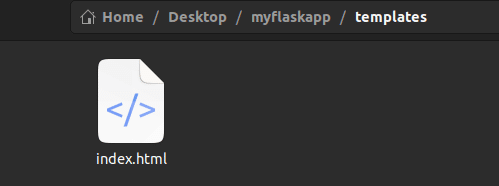
In app.py
, import the render_template
function from Flask and update the home()
function to render the index.html
template:
from flask import Flask,render_template app = Flask(__name__) @app.route('/') def home(): return render_template('index.html') # return "Welcome to my Flask web app!" @app.route('/about') def about(): return "This is my Flask web app!"
Now, when users visit http://127.0.0.1:5000/
, they will see the content of the index.html
template.
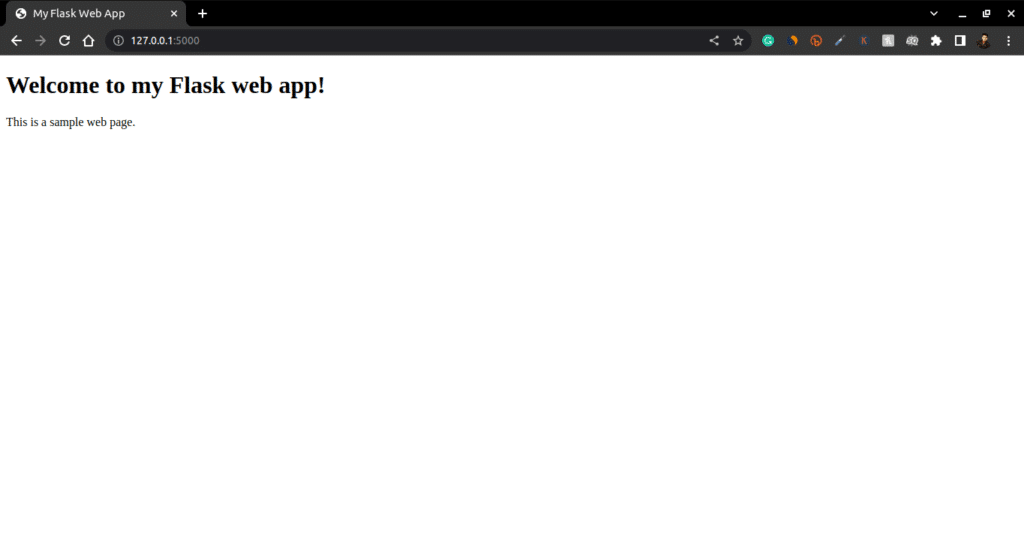
Video Tutorial
Conclusion
Congratulations on successfully building your first web app using Flask! In this article, we guided you through the process of setting up Flask, constructing a basic web app, running the app, expanding its functionality, and implementing templates. With this solid foundation, we encourage you to explore Flask’s documentation and experiment with different features to enhance your web app further.
So in this way, you can create your first web app using Flask. Check out my Flask projects here.
Read my last article – 20+ Top Machine Learning Projects for final year
Check out my other machine learning projects, deep learning projects, computer vision projects, NLP projects, Flask projects at machinelearningprojects.net