Hey guys in this blog we will build a Drowsiness Detection app that will detect if the person in the video is getting Drowsy or not.
This is a very interesting yet easy project with even less than 80 lines of code, so without any further due, let’s do it…
Sneak at our Output
Code for Drowsiness Detection
from imutils import face_utils import dlib import cv2 from pygame import mixer thres = 6 mixer.init() sound = mixer.Sound('alarm.wav') dlist = [] detector = dlib.get_frontal_face_detector() predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat") cap = cv2.VideoCapture(0) def dist(a,b): x1,y1 = a x2,y2 = b return ((x1-x2)**2 + (y1-y2)**2)**0.5 while True: # Getting out image by webcam _, image = cap.read() # Converting the image to gray scale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Get faces into webcam's image rects = detector(gray, 0) # For each detected face, find the landmark. for (i, rect) in enumerate(rects): # Make the prediction and transfom it to numpy array shape = predictor(gray, rect) shape = face_utils.shape_to_np(shape) # Draw on our image, all the finded cordinate points (x,y) for (x, y) in shape: cv2.circle(image, (x, y), 2, (0, 255, 0), -1) le_38 = shape[37] le_39 = shape[38] le_41 = shape[40] le_42 = shape[41] re_44 = shape[43] re_45 = shape[44] re_47 = shape[46] re_48 = shape[47] dlist.append((dist(le_38,le_42)+dist(le_39,le_41)+dist(re_44,re_48)+dist(re_45,re_47))/4<thres) if len(dlist)>10:dlist.pop(0) # Drowsiness detected if sum(dlist)>=4: try: sound.play() except: pass else: try: sound.stop() except: pass # Show the image cv2.imshow("Output", image) if cv2.waitKey(5) & 0xFF == 27: break cv2.destroyAllWindows() cap.release()
- Line 1-4: Importing required libraries.
- Line 6: Setting a threshold (will see in the code ahead).
- Line 8-9: Creating alarm sound using the pygame module to use it further in the app.
- Line 11: An empty list that we will use ahead.
- Line 13: Using dlib face detector.
- Line 14: Using dlib landmarks detector to detect eye points.
- Line 16: Initializing cap object to use Webcam later.
- Line 18-21: A simple distance function that calculates the distance between two coordinates.
- Line 25: Reading images from the webcam.
- Line 27: Converting them to Grayscale.
- Line 30: Detecting faces.
- Line 33: Start traversing in those faces.
- Lin 35-36: Get the 68 face landmarks and convert them to a NumPy array.
- Line 39-40: Draw all the landmarks.
- Line 42-50: Extract required eye landmarks.
- For the left eye, we will extract 38, 39, 42, and 41.
- For the right eye, we will extract 44, 45, 48, and 47.
- Read the algo below once before reading further steps.
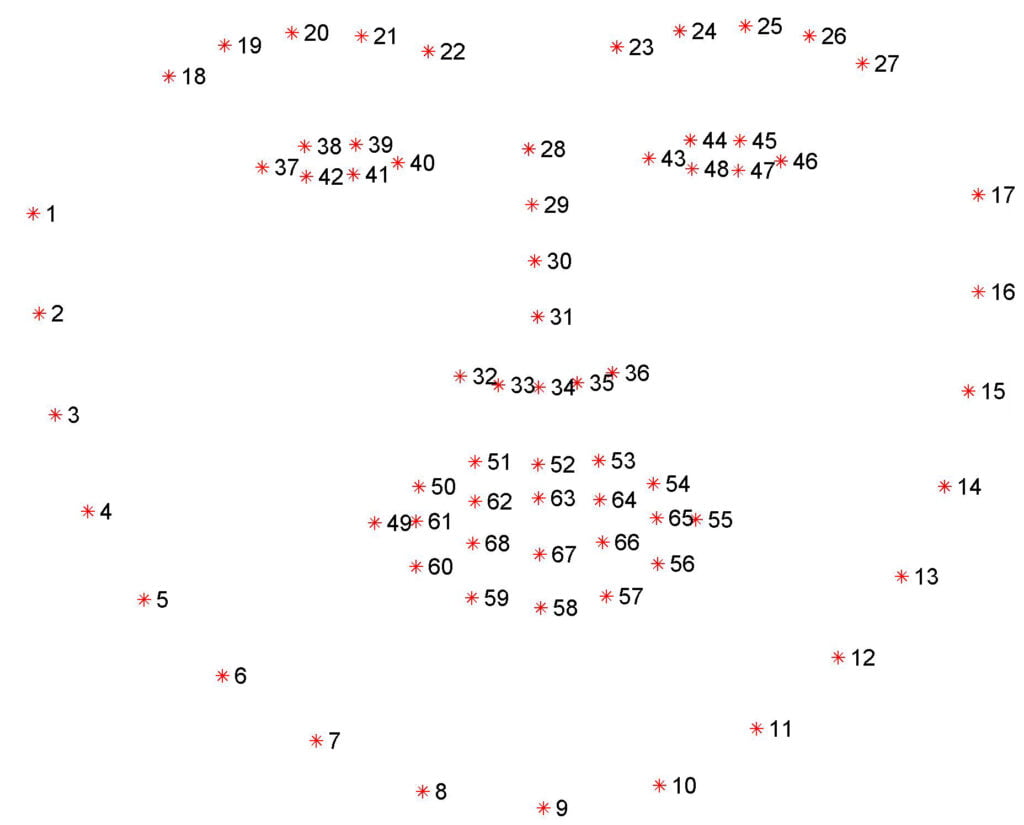
- Line 54: This line is responsible for keeping the size of dlist=10.
- Deletion is done from the front and addition is done in end.
- It’s like a queue system.
- Line 57-66: Play the alarm, when there are at least 4 Trues in the dlist. Stop it when they are less than 4.
- Line 69 – Show the Image.
- Line 71-72: Stop the app when the user hits the ESC key.
- Line 74-75: Close all cv2 windows and release the webcam.
Algo for detecting Drowsiness:
- Find the distances between 38-42, 39-41, 44-48, and 45-47 landmarks and find the average of those 4.
- If this average is greater than thres we defined initially, append/add a True in our dlist.
- If our dlist contains 4 or more Trues, it means in continuous 4 frames, drowsiness is detected so play the alarm.
- We have kept this concept of 4 or more because if we don’t do so, our program will play an alarm even when we blink which is False Positive.
Output
Download Source Code
Other Ideas for Performing Drowsiness Detection:
- One way is what we performed above.
- The second way can be to train a Neural Network on face images.
- The third way can be to train a Neural Network on the eyes images.
So this is how you can perform Drowsiness Detection in python using cv2 and dlib.
Check out my other machine learning projects, deep learning projects, computer vision projects, NLP projects, and Flask projects at machinelearningprojects.net