In today’s blog, we are going to perform one of the most important operations of image processing which is thresholding. So without any further due, let’s do it…
Step 1 – Import the libraries required for thresholding.
import cv2 import matplotlib.pyplot as plt
Step 2 – Read the grayscale image.
img = cv2.imread('gray21.512.tiff')
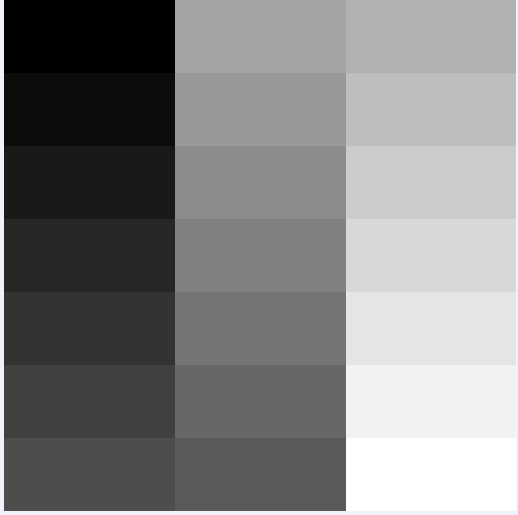
Step 3 – Let’s instantiate some values.
th = 127 max_val = 255
- Here we have set our threshold value to 127.
- Also, we have set our max value to 255.
Step 4 – Performing the thresholding operation using the following 5 methods.
ret, o1 = cv2.threshold(img, th, max_val, cv2.THRESH_BINARY) ret, o2 = cv2.threshold(img, th, max_val, cv2.THRESH_BINARY_INV) ret, o3 = cv2.threshold(img, th, max_val, cv2.THRESH_TOZERO) ret, o4 = cv2.threshold(img, th, max_val, cv2.THRESH_TOZERO_INV) ret, o5 = cv2.threshold(img, th, max_val, cv2.THRESH_TRUNC)
- cv2.threshold() returns 2 values, first is the return value(True or False) and second is the output image.
- cv2.THRESH_BINARY method gives the max value as soon as the value crosses the threshold.
- cv2.THRESH_BINARY_INV method gives max value till it does not crosses the threshold.
- cv2.THRESH_TOZERO method gives a 0 value till it does not crosses the threshold.
- cv2.THRESH_TOZERO_INV method gives 0 as it crosses the threshold.
- cv2.THRESH_TRUNC method gives a threshold value as it crosses the threshold.
NOTE – Refer to the resulting image below for further understanding.
Step 5 – Plot the results.
output = [img, o1, o2, o3, o4, o5] titles = ['Original', 'Binary', 'Binary Inv', 'Zero', 'Zero Inv', 'Trunc'] for i in range(6): plt.subplot(2, 3, i + 1) plt.imshow(output[i]) plt.title(titles[i]) plt.xticks([]) plt.yticks([]) plt.show()
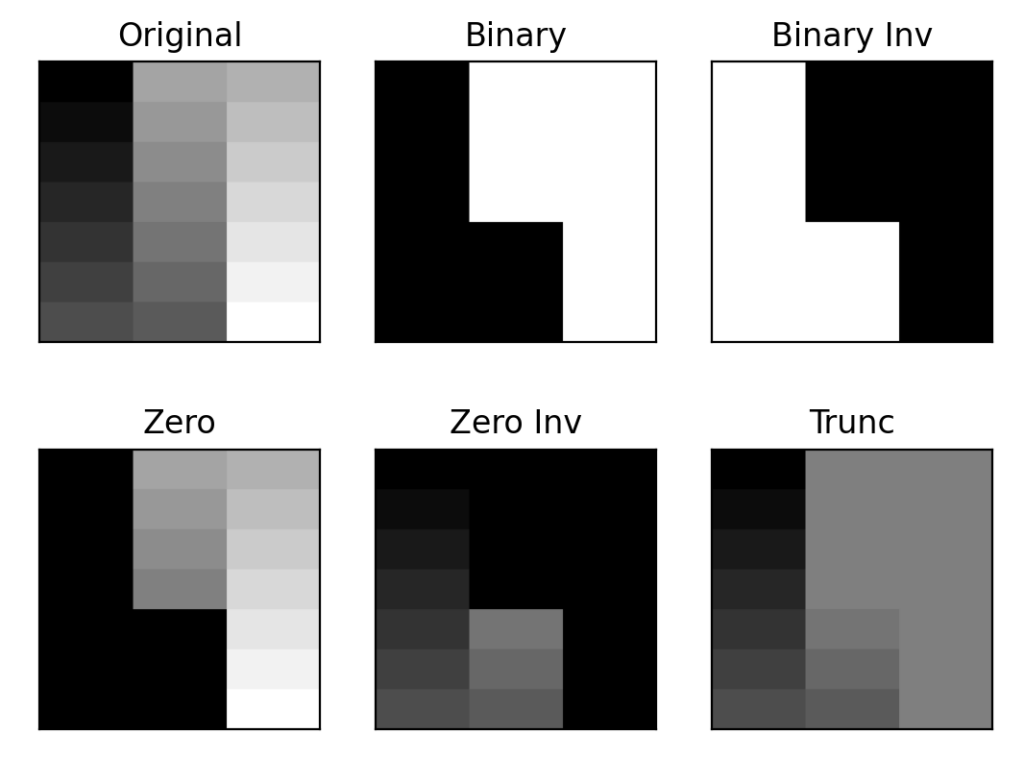
NOTE – Thresholding can be further optimized by using OTSU Thresholding.
ret, o1 = cv2.threshold(img, 0, max_val, cv2.THRESH_BINARY+cv2.THRESH_OTSU) ret, o2 = cv2.threshold(img, 0, max_val, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU) ret, o3 = cv2.threshold(img, 0, max_val, cv2.THRESH_TOZERO+cv2.THRESH_OTSU) ret, o4 = cv2.threshold(img, 0, max_val, cv2.THRESH_TOZERO_INV+cv2.THRESH_OTSU) ret, o5 = cv2.threshold(img, 0, max_val, cv2.THRESH_TRUNC+cv2.THRESH_OTSU)
- Simply just add a cv2.THRESH_OTSU as a flag as shown above to implement it.
- The main advantage that we have with OTSU is that in this method we don’t need to specify the threshold value (just specify 0 there) like in previous methods(without the OTSU method), it determines it automatically.
Let’s see the whole code…
import cv2 import matplotlib.pyplot as plt img = cv2.imread('gray21.512.tiff') th = 127 max_val = 255 # give max value as soon as value crosses threshold ret, o1 = cv2.threshold(img, th, max_val, cv2.THRESH_BINARY) # give max value till it does not crosses threshold ret, o2 = cv2.threshold(img, th, max_val, cv2.THRESH_BINARY_INV) # give 0 value till it does not crosses threshold ret, o3 = cv2.threshold(img, th, max_val, cv2.THRESH_TOZERO) # give 0 as it crosses threshold ret, o4 = cv2.threshold(img, th, max_val, cv2.THRESH_TOZERO_INV) # give threshold value as it crosses threshold ret, o5 = cv2.threshold(img, th, max_val, cv2.THRESH_TRUNC) output = [img, o1, o2, o3, o4, o5] titles = ['Original', 'Binary', 'Binary Inv', 'Zero', 'Zero Inv', 'Trunc'] for i in range(6): plt.subplot(2, 3, i + 1) plt.imshow(output[i]) plt.title(titles[i]) plt.xticks([]) plt.yticks([]) plt.show()
NOTE – Read more about thresholding operation here.
Do let me know if there’s any query regarding this topic by contacting me by email or LinkedIn.
So this is all for this blog folks, thanks for reading it and I hope you are taking something with you after reading this and till the next time ?…
Read my previous post: HOW TO SPLIT AND MERGE CHANNELS IN PYTHON USING OPENCV
Check out my other machine learning projects, deep learning projects, computer vision projects, NLP projects, Flask projects at machinelearningprojects.net.