Question
A binary tree is uni-valued if every node in the tree has the same value.
Given the root
of a binary tree, return true
if the given tree is uni-valued, or false
otherwise.
Example 1:
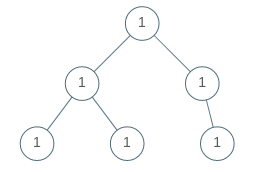
Input: root = [1,1,1,1,1,null,1] Output: true
Example 2:
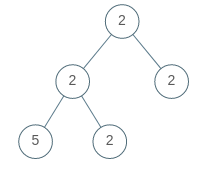
Input: root = [2,2,2,5,2] Output: false
Constraints:
- The number of nodes in the tree is in the range
[1, 100]
. 0 <= Node.val < 100
Python Solution
# Definition for a binary tree node. # class TreeNode: # def __init__(self, val=0, left=None, right=None): # self.val = val # self.left = left # self.right = right class Solution: def isUnivalTree(self, root: TreeNode) -> bool: stack = [root] prev = root.val while len(stack)!=0: x = stack.pop() if x==None: continue curr = x.val if prev!=curr: return False stack.append(x.left) stack.append(x.right) prev = curr return True